MARCIN.com
Marcin Jamro, PhD, DSc
How to compute MD5 or SHA-512 hash from string?
C# | .NET
Both MD5 and SHA-512 are hashing algorithms that use the cryptographic hash function to compute a hash (also referred as a digital fingerprint or a checksum) representing input data. A hash is of a specific size, such as 128 (in the case of MD5) or 512 bits (for SHA-512). A hash algorithm can be used to convert a user's password into the form from which it cannot be restored. While the user is logging in to the system, you need to generate a hash of the entered password and check whether it is the same as the hash stored in the database. Thanks to this approach, you do not store plain passwords. To even further increase security of stored passwords, it is a good practice to use additional data, named salt. Another example is to get a checksum of a large file and compare it with the provided hash on the download size to ensure that the file is downloaded correctly and there is no data corruption. In the case of hashing algorithms, small changes in the input data result in significant and unpredictable changes in the hash. Thus, you can easily discover differences between files.
The first hashing algorithm is named MD5. The hash size for it is only 128 bits and due to known collision problems, this algorithm should not be used in real-world scenarios. However, as this algorithm has a long history and was popular, it is worth noting it and you can find a lot of information about it. If you want to compute a hash of a byte array, you can use the following code:
using MD5 md5 = MD5.Create(); byte[] inputBytes = Encoding.UTF8.GetBytes("P@s$w0rD"); byte[] hashBytes = md5.ComputeHash(inputBytes); string hash = GetString(hashBytes);
At the beginning, you create a new instance of the class representing the MD5 algorithm. Then, you get a byte array from the input string. Computing a hash is performed by calling the ComputeHash
method. It returns a byte array with 16 elements, which you can convert into string using the auxiliary method:
string GetString(byte[] bytes) { StringBuilder sb = new(); foreach (byte i in bytes) { sb.Append($"{i:x2}"); } return sb.ToString(); }
When you run the code, you can see the following result:
55dfb973e6d8f7126fa3a914822cfe00
The received hash is quite short, so - once again - please remember to use other hashing algorithm in your applications. One of the candidates is SHA-512, which is a much safer solution, and its hash size is 512 bits, so a byte array contains 64 elements. The code for computing the hash is almost the same, but another class is used, namely SHA512
, as follows:
using SHA512 sha = SHA512.Create(); byte[] inputBytes = Encoding.UTF8.GetBytes("P@s$w0rD"); byte[] hashBytes = sha.ComputeHash(inputBytes); string hash = GetString(hashBytes);
The generated hash is 4 times longer than in MD5. The hash for the same input (namely P@s$w0rD
) is presented below:
629ccfe628b952b098b9f881cf24b726 2d2c7b119a3bc36a8cc0190e8c8b82af 80f642bb4c756036c4b6cdb5471afcb2 3d550ce1b7928483ceaebf85d8983c7e
The content, including any part of code, is presented without warranty, either express or implied. The author cannot be held liable for any damages caused or alleged to have been caused directly or indirectly by any content shown on this website.
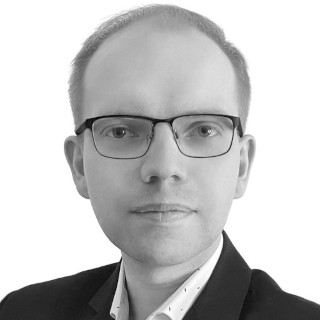
Hello, I am Marcin
Reliable entrepreneur with 10+ years of companies operation, such as CEO at a few IT companies. I was an author of a few software & hardware products, still open to new ideas & cooperation.
Helpful expert with 10+ years of experience, together with PhD and DSc in Computer Science. I was an author of books and publications, as well as an expert in international projects.
Experienced developer with 10+ years of development and 100+ completed projects. I worked on various complex international projects, e.g. at Microsoft in USA and as CTO at a few companies. I have MCP, MCTS and MCPD certificates.
You can read more about me in my short bio. I am waiting for contact at [email protected], as well as at my Facebook and LinkedIn profiles.
Books
I am an author of a few books and numerous publications, also in high-quality international scientific journals.
If you want to learn data structures and algorithms in the context of C#, let's take a look at my newest book. It is the second edition of C# Data Structures and Algorithms. You can buy it here.
Projects
Do you like traveling? If so, discover an amazing world with local guides, right here and right now at: https://camaica.com
Do you follow your favorite creators? If so, check it out what products they recommend and buy them at: https://refspace.com
Travels
I love travels, learning new cultures and regions, meeting outstanding people, as well as taking pictures of beautiful places. Take a look at my travel diary.