MARCIN.com
Marcin Jamro, PhD, DSc
How to encrypt and decrypt text with AES symmetric algorithm?
C# | .NET | Algorithms
An encryption algorithm is used to encrypt data, which means encoding information (plain text) to another form (cipher text) that should be possible to decode by the authorized person only. There are many encryption algorithms, among which two subtypes can be mentioned, namely symmetric-key and asymmetric-key (also called public-key). Encryption is commonly used in various systems, such as for protecting communication in the popular messengers, for the digital rights management systems, or for protecting wireless communication. Currently, it is a standard to secure communication, so the knowledge of the encryption algorithms should be the "must have" for any developer.
A representative of the symmetric-key encryption algorithms is Advanced Encryption Standard, also referred to as AES or Rijndael. As it is a symmetric-key type, the same initialization vector (IV) and key are used for encrypting the plain text into the cipher text and for decrypting the cipher text into the plain text. If a user does not have either IV or key, one's is no able to decrypt the cipher text, as shown in the following illustration:

The application of the AES algorithm is very simple with the usage of the C# language, because it is supported with the abstract Aes
class and other related classes. The way of encrypting a plain text into a byte array is shown as follows:
byte[] Encrypt(string plainText, byte[] key, byte[] iv) { using Aes aes = GetAes(key, iv); ICryptoTransform et = aes.CreateEncryptor(); using MemoryStream ms = new(); using CryptoStream cs = new(ms, et, CryptoStreamMode.Write); using (StreamWriter sw = new(cs)) { sw.Write(plainText); } return ms.ToArray(); }
At the beginning, you create an instance of the class representing the AES algorithm, using the auxiliary GetAes
method, which code is shown below:
Aes GetAes(byte[] key, byte[] iv) { Aes aes = Aes.Create(); aes.Key = key; aes.IV = iv; return aes; }
Here, you use the Create
method of the Aes
class. Then, you set the key and the initialization vector using the values passed as parameters. Then, let's go back to the implementation of the Encrypt
method. In the next lines, you create an encryptor and use two streams, namely MemoryStream
and CryptoStream
. Then, you encrypt data and write them to the MemoryStream
instance. At the end, you just return its content as an array of bytes.
As you can see, encrypting data is very simple and the situation looks similar in the case of decrypting, as follows:
string Decrypt(byte[] cipherBytes, byte[] key, byte[] iv) { using Aes aes = GetAes(key, iv); ICryptoTransform dt = aes.CreateDecryptor(); using MemoryStream ms = new(cipherBytes); using CryptoStream cs = new(ms, dt, CryptoStreamMode.Read); using StreamReader sr = new(cs); return sr.ReadToEnd(); }
Now, let's take a look at the exemplary usage of the AES algorithm:
byte[] iv = GetRandom(16); byte[] key = GetRandom(32); string plainText = "It is a secret message!"; byte[] cipherBytes = Encrypt(plainText, key, iv); string decryptedText = Decrypt(cipherBytes, key, iv);
At the beginning, you generate a random initialization vector and a key. The last two lines allow you to encrypt text into an array of bytes and then to decrypt the array of bytes into the text. As you see, for both encryption and decryption, the same IV and key are required.
By the way, AES is not the only symmetric-key algorithm. Among other, you can find Blowfish, Twofish, or Triple DES.
The content, including any part of code, is presented without warranty, either express or implied. The author cannot be held liable for any damages caused or alleged to have been caused directly or indirectly by any content shown on this website.
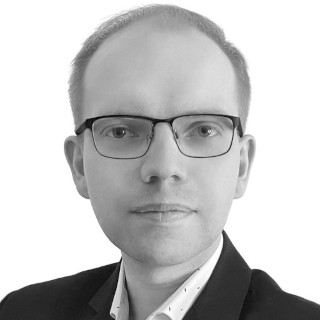
Hello, I am Marcin
Reliable entrepreneur with 10+ years of companies operation, such as CEO at a few IT companies. I was an author of a few software & hardware products, still open to new ideas & cooperation.
Helpful expert with 10+ years of experience, together with PhD and DSc in Computer Science. I was an author of books and publications, as well as an expert in international projects.
Experienced developer with 10+ years of development and 100+ completed projects. I worked on various complex international projects, e.g. at Microsoft in USA and as CTO at a few companies. I have MCP, MCTS and MCPD certificates.
You can read more about me in my short bio. I am waiting for contact at [email protected], as well as at my Facebook and LinkedIn profiles.
Books
I am an author of a few books and numerous publications, also in high-quality international scientific journals.
If you want to learn data structures and algorithms in the context of C#, let's take a look at my newest book. It is the second edition of C# Data Structures and Algorithms. You can buy it here.
Projects
Do you like traveling? If so, discover an amazing world with local guides, right here and right now at: https://camaica.com
Do you follow your favorite creators? If so, check it out what products they recommend and buy them at: https://refspace.com
Travels
I love travels, learning new cultures and regions, meeting outstanding people, as well as taking pictures of beautiful places. Take a look at my travel diary.